Hello! This a tutorial to make a trap for your players. when they step on it they will need to make a Saving throw. There is a bunch of different methods to do this, but this one will automate the process.
To get started you’ll need these modules:
Dynamic Effects.
Minor-QOL.
Furnace.
Trigger Happy.
Make sure you have them all installed and enabled. Make sure you also have a Trigger Happy
Journal Entry or Folder.
There are 3 options on how to deal with “Traps”, each one as viable as the other.
Example 1 - Semi-Automated (Asks players to make a roll)
Example 2 - Fully Automated (Rolls for a player)
Example 3 - Pauses The Game. Can add Chat message**
Example 1 - Semi Automated
For this option, You’ll need The module: LMRTFY (Let me roll that for you)
Macro
To start off, make a macro.
Name it: DoTrapAttack
Make sure it is set to: Script
Disable Execute Macro As GM
Minor-QOL Macro
let tactor = game.actors.entities.find(a => a.name === args[0])
if (!tactor) return `/Whisper GM "DoTrap: Target token ${args[0]} not found"`
let item = tactor.items.find(i=> i.name === args[1])
if (!item) return `/Whisper GM "DoTrap: Item ${args[1]} not found"`
let oldTargets = game.user.targets;
game.user.targets = new Set().add(token);
Hooks.once("MinorQolRollComplete", () => {
MinorQOL.forceRollDamage=false;
game.user.targets=oldTargets;
})
MinorQOL.forceRollDamage = true;
await MinorQOL.doCombinedRoll({actor, item, event, token})
let trapToken = canvas.tokens.placeables.find(t=>t.name === args[2])
if (trapToken) await DynamicEffects.setTokenVisibility(trapToken.id, true);
Midi-QOL Macro
// Requires midi-qol to work at all
// Rolls an attack from actor args[0] using item args[1] and display a token args[2]
let tactor = game.actors.entities.find(a => a.name === args[0])
if (!tactor) return `/Whisper GM "DoTrap: Target token ${args[0]} not found"`
let item = tactor.items.find(i=> i.name === args[1])
if (!item) return `/Whisper GM "DoTrap: Item ${args[1]} not found"`
let trapToken = canvas.tokens.placeables.find(t=>t.name === args[2])
new MidiQOL.TrapWorkflow(tactor, item, [token], trapToken.center)
Save it.
Note: Nothing in this macro should be changed, other than the name.
Character, Token & Weapons
First character you have to create, is where the “Trap Damage/Saving Throw” is coming from. This needs to be an actor.
Create an actor, in this example, we will call it Traps
.
Go inside this actors inventory, and create an Item, in this example, we’ll call it Spikes
When making this item, go to details.
Action Type → Saving throw
Here you can mess around with the different settings, set how much damage etc.
For mine, I’ve made it into a sort of Pit Trap with spikes. They’ll have to make a dexterity saving throw against the DC of 15. Just set it to flat unless you want something special.
Important: You can make all the items on this character.
Now we’ll make the actual trap, so you can set those nice trap icons from 2minutetabletop.
Make a new character, I’ll call this actor Spike Trap
. Set my actor token picture, and all of that nice stuff
Then drag it onto the board where you want the trap to be. On the board Double Right-click
the token, and rename it. In my case, I’ll name it Trap4
.
Make sure that it is not linked.
Update the token.
Now Hide the token.
Trigger Happy
Now Trigger Happy, open your Journal and you should do something along these lines(ctrl-shift-v to clear formatting on paste):
@Token[Trap4] @Trigger[capture move] @ChatMessage[/DoTrapAttack Traps "Spikes" Trap4]
Where:
Token[Trap4]
is the token of the trap.
@Trigger[capture move]
is “when this token is landed/walked on” - “When Trap4 is landed/walked on”
Do:
@chatMessage[/DoTrapAttack Traps "Spikes" Trap4
DoTrapAttack
is the macro we made.
Traps
is the Character that holds thetrap items
.
"Spikes"
is the name of the Item it will use to determine damage and saving throw. Yes, it needs"
"
.
Trap4
on the end is what token to Unhide, in this case, it wants to reveal itself.
Settings
Last thing you’ll need to do, is just enable go into Minor-QOL settings, and set Prompt Players to Roll Saves
to Let Me Roll That For You
Example 2 (Fully Automated)
Macro
To start off, make a macro.
Name it: DoTrapAttack
Make sure it is set to: Script
Disable Execute Macro As GM
Minor-QOL Macro
let tactor = game.actors.entities.find(a => a.name === args[0])
if (!tactor) return `/Whisper GM "DoTrap: Target token ${args[0]} not found"`
let item = tactor.items.find(i=> i.name === args[1])
if (!item) return `/Whisper GM "DoTrap: Item ${args[1]} not found"`
let oldTargets = game.user.targets;
game.user.targets = new Set().add(token);
Hooks.once("MinorQolRollComplete", () => {
MinorQOL.forceRollDamage=false;
game.user.targets=oldTargets;
})
MinorQOL.forceRollDamage = true;
await MinorQOL.doCombinedRoll({actor, item, event, token})
let trapToken = canvas.tokens.placeables.find(t=>t.name === args[2])
if (trapToken) await DynamicEffects.setTokenVisibility(trapToken.id, true);
Midi-QOL Macro
// Requires midi-qol to work at all
// Rolls an attack from actor args[0] using item args[1] and display a token args[2]
let tactor = game.actors.entities.find(a => a.name === args[0])
if (!tactor) return `/Whisper GM "DoTrap: Target token ${args[0]} not found"`
let item = tactor.items.find(i=> i.name === args[1])
if (!item) return `/Whisper GM "DoTrap: Item ${args[1]} not found"`
let trapToken = canvas.tokens.placeables.find(t=>t.name === args[2])
new MidiQOL.TrapWorkflow(tactor, item, [token], trapToken.center)
Save it.
Note: Nothing in this macro should be changed, other than the name.
Character, Token & Weapons
First character you have to create, is where the “Trap Damage/Saving Throw” is coming from. This needs to be an actor.
Create an actor, in this example, we will call it Traps
.
Go inside this actors inventory, and create an Item, in this example, we’ll call it Spikes
When making this item, go to details.
Action Type → Saving throw
Here you can mess around with the different settings, set how much damage etc.
For mine, I’ve made it into a sort of Pit Trap with spikes. They’ll have to make a dexterity saving throw against the DC of 15. Just set it to flat unless you want something special.
Important: You can make all the items on this character.
Now we’ll make the actual trap, so you can set those nice trap icons from 2minutetabletop.
Make a new character, I’ll call this actor Spike Trap
. Set my actor token picture, and all of that nice stuff
Then drag it onto the board where you want the trap to be. On the board Double Right-click
the token, and rename it. In my case, I’ll name it Trap4
.
Make sure that it is not linked.
Update the token.
Now Hide the token.
Trigger Happy
Now Trigger Happy, open your Journal and you should do something along these lines(ctrl-shift-v to clear formatting on paste):
@Token[Trap4] @Trigger[capture move] @ChatMessage[/DoTrapAttack Traps "Spikes" Trap4]
Where:
Token[Trap4]
is the token of the trap.
@Trigger[capture move]
is “when this token is landed/walked on” - “When Trap4 is landed/walked on”
Do:
@chatMessage[/DoTrapAttack Traps "Spikes" Trap4
DoTrapAttack
is the macro we made.
Traps
is the Character that holds thetrap items
.
"Spikes"
is the name of the Item it will use to determine damage and saving throw. Yes, it needs"
"
.
Trap4
on the end is what token to Unhide, in this case, it wants to reveal itself.
Settings
This will by default be automated.
If it isn’t, go into Minor-QOL settings and set Prompt Players to Roll Saves
to chat
Example 3 - Pause Game
You’ll not need Minor QOL for this to work.
Macro
To pause the game when a trap is stepped on, and reveal the token you’ll need this macro:
Reveal Trap Macro:
Name it: RevealTrap
Make sure it is set to: Script
Enable Execute Macro As GM
game.togglePause(true, true);
let trapToken = canvas.tokens.placeables.find(t=>t.name === args[0])
if (trapToken) await DynamicEffects.setTokenVisibility(trapToken.id, true);
Note: If you don’t want to pause, delete game.togglePause(true, true);
Token
Now we just need to make the trap token. First create a character, for this I’ll call it Bear Trap
Customize the token to what picture you like, I’ll give mine a neat Beartrap look.
Drag it onto the board. Now we’ll double right-click
the token on the board to rename it. For this tutorial, I’ll call it BearTrap1
.
Make sure that it is not linked.
Update The Token.
Trigger Happy
In Trigger Happy, you’ll just need to specify the name of the token you’ve made.
Mine looks like(ctrl-shift-v to clear formatting on paste):
@Token[BearTrap1] @Trigger[capture move] @ChatMessage[/RevealTrap BearTrap1] @ChatMessage[Someone Stepped in a trap!]
Where:
Token[BearTrap1]
is the token of the trap.
@Trigger[capture move]
is “when this token is landed/walked on” - “WhenBearTrap1
is landed/walked on”
Do:
@ChatMessage[/RevealTrap BearTrap1]
WhereBearTrap1
is the trap we want to reveal.
@ChatMessage[Someone Stepped in a trap!]
is giving the chat message “Someone Stepped in a trap!” in chat.
Troubleshooting
Folder
If you’re using a folder
for Trigger Happy, that the Journal you’re using is not named “Trigger Happy”. This will cause it to trigger twice, and make some weird bugs. Just re-name the Journal Entry.
The Folder or Journal Entry is case sensitive. Make sure it is Capital T
and H
in Trigger Happy
.
Token
Make sure that you’ve edited the Token
Name. It doesn’t need to represent anything. Also, make sure that the Token is Not Linked
.
Currently known Bugs:
Weapons
You should leave flavour text in the weapons empty. It might cause issues to the item.
If you want flavour text, either make a macro, or do @Chatmessage[YOU FOOL, STEPPED IN MY TRAP]
in Trigger Happy.
GM & Players
There is currently a bug with what order people join the game in. Recommended is for the GM to join first, then the rest of the players. If there is multiple GM’s, it may cause problems sadly.
There have been a couple of issues with traps and minor-qol saving rolls.
The not applying damage issue should only be an issue when there was more than 1 dm logged in and then only for one of the dm clients. That should now be fixed.
The not rolling saving throws issue is (I think) related to clients not rgistering as logged on the dm client. That is a problem since minor-qol checks to see that the player is active (i.e. logged in and connected to the gm client) before using LMRTFY to roll saves.
When you notice saves not being rolled can you checck the player list and see if the player is listed on the gm client. I have noticed that refreshing the clients in the wrong order can cause the player not to be recognised by the gm client. The better order for refresh is GM first, wait for that to complete, then player, then check the client list on the gm.
One last thing to mention. The save is only requested for the “primary” (or whatever the word is) character for the player - the one listed in brackets after the player on the player list.
@tposney
Settings
I would recommend these settings:
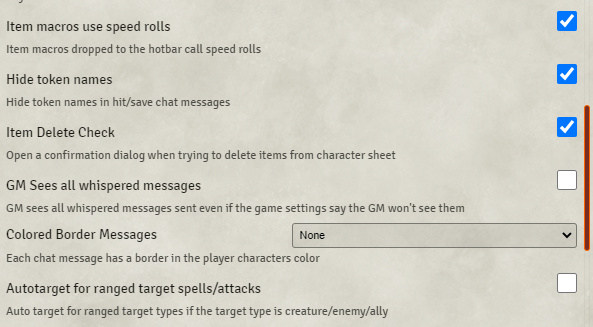
Got any other issues?
You can either leave a comment here, in Kakarotos Discord Server, or in Foundrys #modules
. Just ping @Kevin-#0001.